Real-Life Use-Cases for useCallback and useMemo
One of my friends gives his react interview, and tell me, his interviewer asks him to give some real-life use-cases where he actually uses the useCallback and useMemo to solve his problem. And unfortunately, he got stuck at that point.
I have also taken lots of interviews and personally observed that developers know the definition of useCallback and useMemo but they actually don’t know when to use them. So they didn’t use it to solve their real-life problems.
So in this blog, I will explain when to use them and solve some real-life use-cases in a very simplified way.
Take a cup of coffee or anything you want 😉 and let’s begin.

useCallback
One of my friends was making arguing with me that we have to wrap the all function in useCallback which we are passing as props in the child component.
“Every callback function should be memoized to prevent useless re-rendering of child components that use the callback function” was the reasoning behind his opinion.
But this reason is so far from the actual truth because we have to use the useCallback when there is complex calculation or rendering of the DOM/ react-component in the child component.
Let me explain with some code.
As you can see in the code whenever we click on the increase count button the child component is getting rerender. And on every time child component paint the 1k div which really affect the performance right.
But if the child component doesn’t contain any complex logic or rendering then is no problem with that.
Now how can we prevent the rendering of the child component? We just need to do a small change. At line no 6 wrap the function getValue value into useCallback with [] dep.
const getValue = useCallback((value) => console.log(value), []);
And this is also the actual use-case for the useCallback because whenever you have to render the huge list. of data, in that case, you have to be careful about the re-render.
useMemo
We have to use the useMemo to store the value which has some complexity to get and which does affect the performance.
Let’s play with some code.
As you can see in the code when you update the count the reducerVallue will get recompute. which badly affects the performance.
Just think if the array length is 10k 🤔.
Output:

So to prevent it you just have to change line no 7 with the following one.
const reducedValue = React.useMemo(() => array.reduce((a,b) =>{console.log('computed again') return a + b;}), [])
Pretty simple right 😎😎.
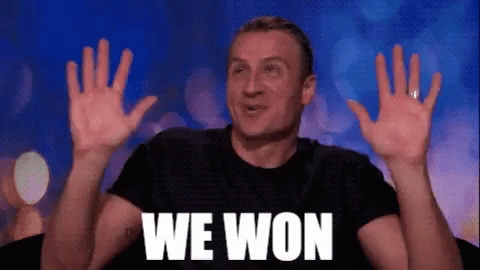
Create ShowMoreTextToggler
I was working on a project where I need to create show more content Components for the description in react-native.

Let me create the same component in ReactJs.
https://gist.github.com/bytecodepandit/7c0db5a93079cfc244394fd0b7f22c20#file-showmoretexttoggler-jsx
As you can see in the code if don’t use the useMemo then in every render it re-computes the value. And computation could be more complex which will affect the performance.
You can also watch my youtube video to make the concept better.
You can find the source code here.
Conclusion
We learn when and how to use useCallback and useMemo. Also solved some real-life use-cases.
Thanks for reading 🙏✌️.
Comments
Post a Comment